安装 faiss
pip3 install faiss
如果报错 ImportError: No module named ‘_swigfaiss 则还需要安装扩展
sudo apt-get install libopenblas-dev sudo apt-get install libomp-dev
简单使用
创建实验用的数据
import numpy as np import faiss import time import pickle d = 5 # 每组中有多少条数据 nb = 200 # 基础数据多少组数据 nq = 100 # 搜索数据有多少组 np.random.seed(1234) # 设置相同的seed,则每次生成的随机数也相同 # xb[:, 0] 取出下标为0的所有元素组成新的列表 # np.arange(nb) 生成从0到200的列表 xb = np.random.random((nb, d)).astype('float32') xb[:, 0] += np.arange(nb) / 1000. xq = np.random.random((nq, d)).astype('float32') xq[:, 0] += np.arange(nq) / 1000.
训练模型、为向量集构建IndexFlatL2索引,其他索引类型在最下面
%time index = faiss.IndexFlatL2(d) # build the index print(index.is_trained) index.add(xb) # add vectors to the index print(index.ntotal)
进行搜索
k = 4 # 查询最相近的4个元素 %time D, I = index.search(xb[:5], k) # 搜索 print(xb[:5]) print(I) # 向量索引位置 print(D) # 相似度矩阵
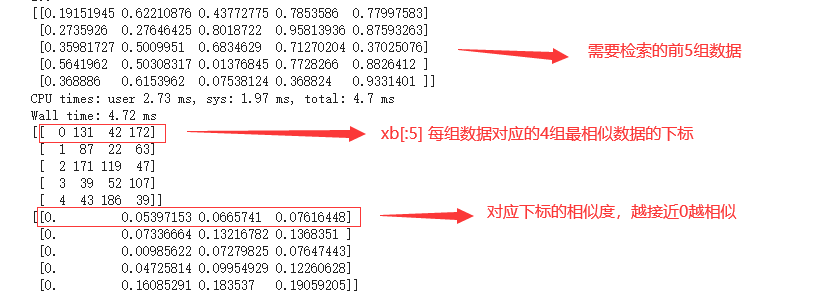

可以看到下标 0 对应的列表和 xb[:5] 的第一组列表完全一致,所以相似度为 0,也就是一样,下标 131 则为第二相似
保存和读取已经创建好的 faiss 索引
faiss.write_index(index, '/content/test.pkl') %time index = faiss.read_index('/content/test.pkl')
读取索引之后,如果需要追加 矩阵数组 到索引里面,直接使用 add 即可
如果需要删除则需要使用 remove_ids 例如删除下标0的列表,需要传入的也是矩阵不是列表
index.remove_ids(np.array([0]))
其他 Faiss 的索引类型
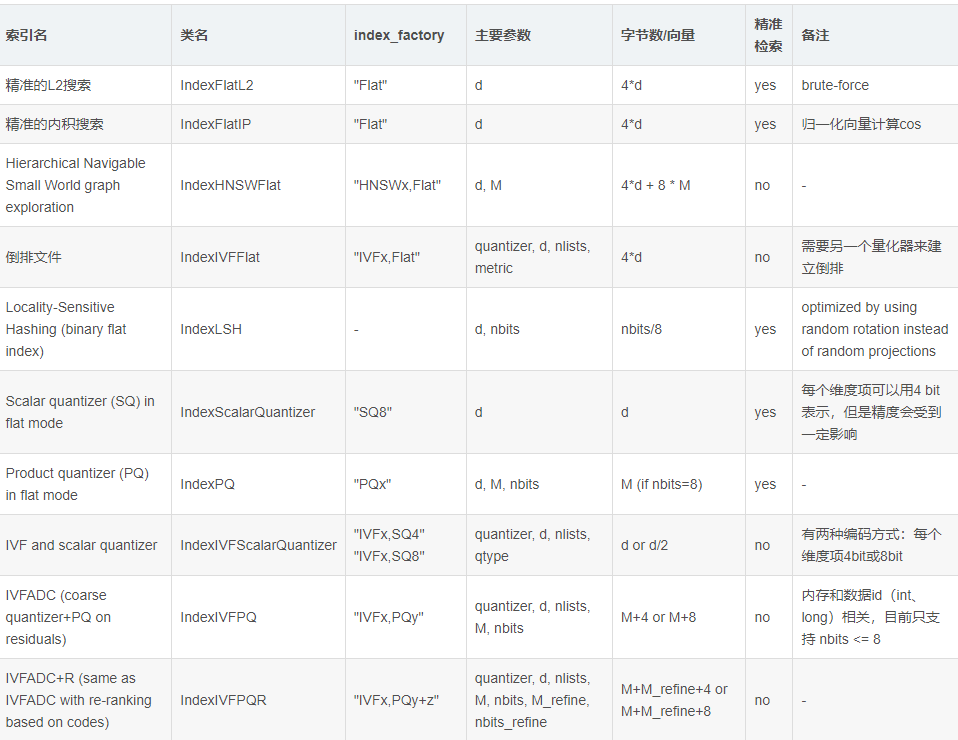
#基于L2距离的确定搜索匹配
#基于内积的确定搜索匹配
#分层索引
#倒排索引
#本地敏感hash
#标量量化索引
#笛卡尔乘积索引
#倒排+标量量化索引
#倒排+笛卡尔乘积索引
#倒排+笛卡尔乘积索引 + 基于编码器重排
评论已关闭。